Debugging your ERC721 NFT metadata
Metadata is a critical part of your token, but debugging issues with it can be challenging as it's often a 'black-box' - it's either working or it's not. We've compiled some common troubleshooting steps to help.
Metadata format
Metadata must follow a specific format to ensure it's displayed the same in every marketplace and wallet. You can read the full spec online, but I've copied the relevant part below.
{
"title": "Asset Metadata",
"type": "object",
"properties": {
"name": {
"type": "string",
"description": "Identifies the asset to which this NFT represents"
},
"description": {
"type": "string",
"description": "Describes the asset to which this NFT represents"
},
"image": {
"type": "string",
"description": "A URI pointing to a resource with mime type image/* representing the asset to which this NFT represents. Consider making any images at a width between 320 and 1080 pixels and aspect ratio between 1.91:1 and 4:5 inclusive."
}
}
}
Validate your metadata
The first step in debugging your metadata should be to validate it, using the OpenSea API. Construct a URI like below with your contract address and a token ID:
Testnets: https://testnets-api.opensea.io/asset/{contractAddress}/{tokenId}/validate/
Mainnet: https://api.opensea.io/asset/{contractAddress}/{tokenId}/validate/
If your metadata is valid you'll get a response with valid: true
, like the below:
{
"valid": true,
"token_uri": "https://concierge.diamondhandshotel.com/ssss/1",
"errors": []
}
If it's not valid, check the errors array for details on why your metadata failed validation.
Force an update
So your metadata is valid, but it's still not showing up? Fetching metadata every time someone views your token could put a lot of demand on your server, so most marketplaces will cache metadata.
You can force an update on OpenSea by adding ?force_update=true
to the end of an asset URL. For example, https://testnets.opensea.io/assets/0xe74cdC48161ba30d38F2E027cB5cb050313d7B03/1?force_update=true
. If you're live on mainnet just remove the testnets
subdomain.
It's also possible to force an update through the UI - just click the refresh icon:
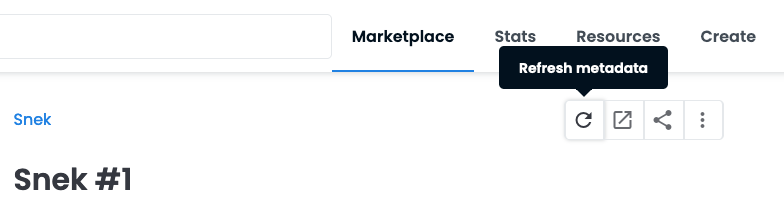
Just wait a bit
NFT marketplaces are under a huge amount of load at the moment due to the rapid growth of the industry. Things can (and do) break, and their metadata service could be down.
Take an early night, and try again in the morning.
Still not working?
Here are some common pitfalls you should look out for:
- Using data: URIs - while some marketplaces support this, it's not officially supported by the spec and may require special attention from the marketplace to enable
- Using data: URIs on OpenSea - OpenSea supports data URIs via the
image_data
key, in which case you should provide a plaintext string (not base 64 encoded). If using SVGs make sure when you serialize to JSON any nested"
are escaped or replaced with'
. - Using an IPFS gateway - if your assets are hosted on IPFS you should use
ipfs://{cid}
URIs directly, don't use a gateway address as they often throttle marketplaces - Serving the correct mime type - your
image
property MUST return a response with animage/*
mimetype. If your token also includes a video, HTML file or other animation you should add the URL to this underanimation_url
- Serving metadata behind Cloudflare - if using Cloudflare you should make sure the security level for your image and metadata endpoints is set to low (otherwise it's possible the metadata bots are getting blocked)